Comparing soundings from NCEP Reanalysis and various models¶
We are going to plot the global, annual mean sounding (vertical temperature profile) from observations.
Read in the necessary NCEP reanalysis data from the online server.
The catalog is here: https://psl.noaa.gov/psd/thredds/catalog/Datasets/ncep.reanalysis.derived/catalog.html
[1]:
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
import xarray as xr
ncep_url = "https://psl.noaa.gov/thredds/dodsC/Datasets/ncep.reanalysis.derived/"
ncep_air = xr.open_dataset( ncep_url + "pressure/air.mon.1981-2010.ltm.nc", decode_times=False)
[2]:
level = ncep_air.level
lat = ncep_air.lat
Take global averages and time averages.
[3]:
Tzon = ncep_air.air.mean(dim=('lon','time'))
weight = np.cos(np.deg2rad(lat)) / np.cos(np.deg2rad(lat)).mean(dim='lat')
Tglobal = (Tzon * weight).mean(dim='lat')
Here is code to make a nicely labeled sounding plot.
[4]:
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + 273.15, np.log(level/1000))
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/1000) )
ax.set_yticklabels( level.values )
ax.set_title('Global, annual mean sounding from NCEP Reanalysis', fontsize = 24)
ax2 = ax.twinx()
ax2.plot( Tglobal + 273.15, -8*np.log(level/1000) );
ax2.set_ylabel('Approx. height above surface (km)', fontsize=16 );
ax.grid()
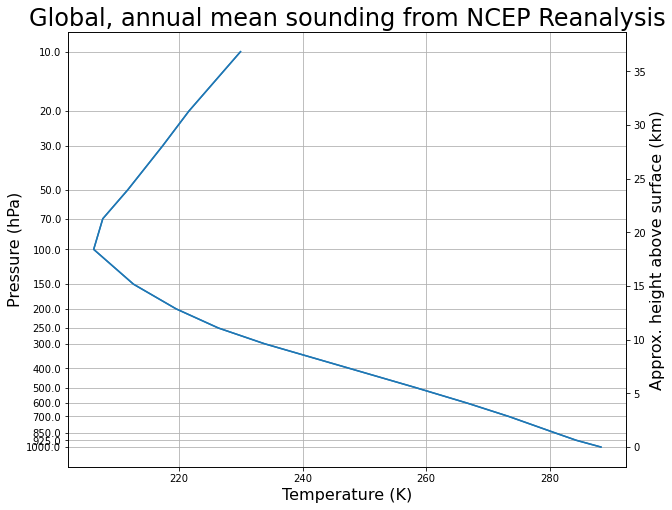
Now compute the Radiative Equilibrium solution for the grey-gas column model¶
[5]:
import climlab
from climlab import constants as const
[6]:
col = climlab.GreyRadiationModel()
print(col)
climlab Process of type <class 'climlab.model.column.GreyRadiationModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.GreyRadiationModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
[7]:
col.subprocess['LW'].diagnostics
[7]:
{'flux_from_sfc': Field([0.]),
'flux_to_sfc': Field([0.]),
'flux_to_space': Field([0.]),
'absorbed': Field([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]),
'absorbed_total': Field([0.]),
'emission': Field([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]),
'emission_sfc': Field([0.]),
'flux_reflected_up': None}
[8]:
col.integrate_years(1)
print("Surface temperature is " + str(col.Ts) + " K.")
print("Net energy in to the column is " + str(col.ASR - col.OLR) + " W / m2.")
Integrating for 365 steps, 365.2422 days, or 1 years.
Total elapsed time is 0.9993368783782377 years.
Surface temperature is [287.84577808] K.
Net energy in to the column is [0.00165505] W / m2.
Plot the radiative equilibrium temperature on the same plot with NCEP reanalysis¶
[9]:
pcol = col.lev
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + 273.15, np.log(level/1000), 'b-', col.Tatm, np.log( pcol/const.ps ), 'r-' )
ax.plot( col.Ts, 0, 'ro', markersize=20 )
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/1000) )
ax.set_yticklabels( level.values )
ax.set_title('Temperature profiles: observed (blue) and radiative equilibrium in grey gas model (red)', fontsize = 18)
ax2 = ax.twinx()
ax2.plot( Tglobal + const.tempCtoK, -8*np.log(level/1000) );
ax2.set_ylabel('Approx. height above surface (km)', fontsize=16 );
ax.grid()
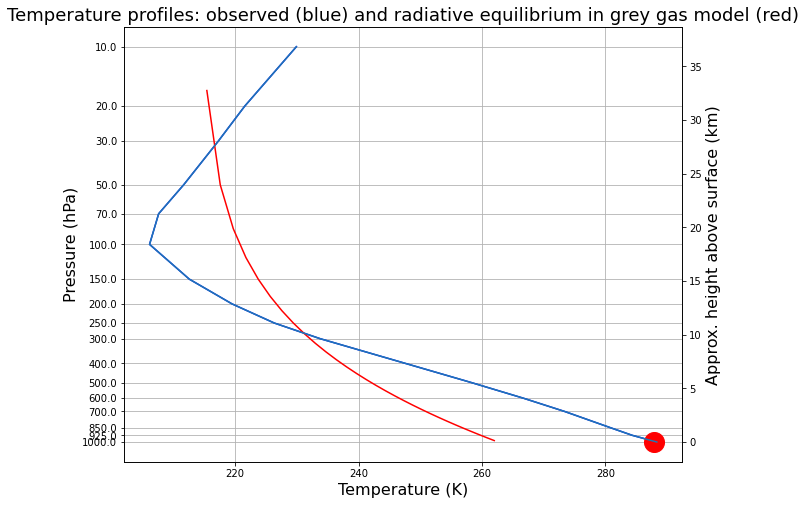
Now use convective adjustment to compute a Radiative-Convective Equilibrium temperature profile¶
[10]:
dalr_col = climlab.RadiativeConvectiveModel(adj_lapse_rate='DALR')
print(dalr_col)
climlab Process of type <class 'climlab.model.column.RadiativeConvectiveModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.RadiativeConvectiveModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
convective adjustment: <class 'climlab.convection.convadj.ConvectiveAdjustment'>
[11]:
dalr_col.integrate_years(2.)
print("After " + str(dalr_col.time['days_elapsed']) + " days of integration:")
print("Surface temperature is " + str(dalr_col.Ts) + " K.")
print("Net energy in to the column is " + str(dalr_col.ASR - dalr_col.OLR) + " W / m2.")
Integrating for 730 steps, 730.4844 days, or 2.0 years.
Total elapsed time is 1.9986737567564754 years.
After 730.0 days of integration:
Surface temperature is [283.040058] K.
Net energy in to the column is [1.09588387e-06] W / m2.
[12]:
dalr_col.param
[12]:
{'timestep': 86400.0,
'water_depth': 1.0,
'albedo_sfc': 0.299,
'Q': 341.3,
'abs_coeff': 0.0001229,
'adj_lapse_rate': 'DALR'}
Now plot this “Radiative-Convective Equilibrium” on the same graph:
[13]:
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + 273.15, np.log(level/1000), 'b-', col.Tatm, np.log( pcol/const.ps ), 'r-' )
ax.plot( col.Ts, 0, 'ro', markersize=16 )
ax.plot( dalr_col.Tatm, np.log( pcol / const.ps ), 'k-' )
ax.plot( dalr_col.Ts, 0, 'ko', markersize=16 )
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/1000) )
ax.set_yticklabels( level.values )
ax.set_title('Temperature profiles: observed (blue), RE (red) and dry RCE (black)', fontsize = 18)
ax2 = ax.twinx()
ax2.plot( Tglobal + const.tempCtoK, -8*np.log(level/1000) );
ax2.set_ylabel('Approx. height above surface (km)', fontsize=16 );
ax.grid()
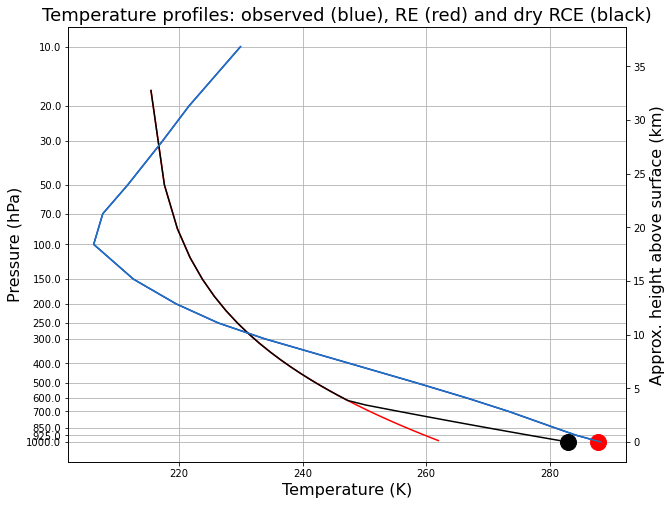
The convective adjustment gets rid of the unphysical temperature difference between the surface and the overlying air.
But now the surface is colder! Convection acts to move heat upward, away from the surface.
Also, we note that the observed lapse rate (blue) is always shallower than \(\Gamma_d\) (temperatures decrease more slowly with height).
“Moist” Convective Adjustment¶
To approximately account for the effects of latent heat release in rising air parcels, we can just adjust to a lapse rate that is a little shallow than \(\Gamma_d\).
We will choose 6 K / km, which gets close to the observed mean lapse rate.
We will also re-tune the longwave absorptivity of the column to get a realistic surface temperature of 288 K:
[14]:
rce_col = climlab.RadiativeConvectiveModel(adj_lapse_rate=6, abs_coeff=1.7E-4)
print(rce_col)
climlab Process of type <class 'climlab.model.column.RadiativeConvectiveModel'>.
State variables and domain shapes:
Ts: (1,)
Tatm: (30,)
The subprocess tree:
Untitled: <class 'climlab.model.column.RadiativeConvectiveModel'>
LW: <class 'climlab.radiation.greygas.GreyGas'>
SW: <class 'climlab.radiation.greygas.GreyGasSW'>
insolation: <class 'climlab.radiation.insolation.FixedInsolation'>
convective adjustment: <class 'climlab.convection.convadj.ConvectiveAdjustment'>
[15]:
rce_col.integrate_years(2.)
print("After " + str(rce_col.time['days_elapsed']) + " days of integration:")
print("Surface temperature is " + str(rce_col.Ts) + " K.")
print("Net energy in to the column is " + str(rce_col.ASR - rce_col.OLR) + " W / m2.")
Integrating for 730 steps, 730.4844 days, or 2.0 years.
Total elapsed time is 1.9986737567564754 years.
After 730.0 days of integration:
Surface temperature is [287.9049635] K.
Net energy in to the column is [2.14745046e-06] W / m2.
Now add this new temperature profile to the graph:
[16]:
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + 273.15, np.log(level/1000), 'b-', col.Tatm, np.log( pcol/const.ps ), 'r-' )
ax.plot( col.Ts, 0, 'ro', markersize=16 )
ax.plot( dalr_col.Tatm, np.log( pcol / const.ps ), 'k-' )
ax.plot( dalr_col.Ts, 0, 'ko', markersize=16 )
ax.plot( rce_col.Tatm, np.log( pcol / const.ps ), 'm-' )
ax.plot( rce_col.Ts, 0, 'mo', markersize=16 )
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/1000) )
ax.set_yticklabels( level.values )
ax.set_title('Temperature profiles: observed (blue), RE (red), dry RCE (black), and moist RCE (magenta)', fontsize = 18)
ax2 = ax.twinx()
ax2.plot( Tglobal + const.tempCtoK, -8*np.log(level/1000) );
ax2.set_ylabel('Approx. height above surface (km)', fontsize=16 );
ax.grid()
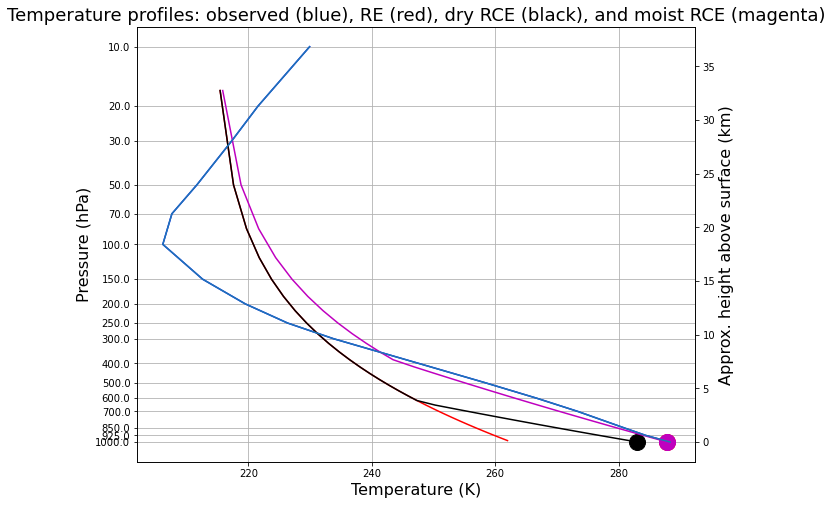
Adding stratospheric ozone¶
Our model has no equivalent of the stratosphere, where temperature increases with height. That’s because our model has been completely transparent to shortwave radiation up until now.
We can load some climatogical ozone data:
[17]:
# Put in some ozone
import xarray as xr
ozonepath = "http://thredds.atmos.albany.edu:8080/thredds/dodsC/CLIMLAB/ozone/apeozone_cam3_5_54.nc"
ozone = xr.open_dataset(ozonepath)
ozone
[17]:
<xarray.Dataset> Dimensions: (lat: 64, lev: 59, lon: 128, time: 12) Coordinates: * lev (lev) float64 0.2842 0.3253 0.3719 ... 849.5 959.0 1.004e+03 * lon (lon) float64 0.0 2.812 5.625 8.438 ... 348.8 351.6 354.4 357.2 * lat (lat) float64 -87.86 -85.1 -82.31 -79.53 ... 82.31 85.1 87.86 * time (time) float64 4.382e+04 4.384e+04 ... 4.412e+04 4.415e+04 Data variables: P0 float64 1.004e+05 date (time) int32 19900116 19900214 19900316 ... 19901115 19901216 datesec (time) int32 0 0 0 0 0 0 0 0 0 0 0 0 OZONE_old (time, lat, lev, lon) float64 ... OZONE (time, lev, lat, lon) float64 ... Attributes: Conventions: NCAR-CSM Source: AMIP II (symmetric for APE project) Written_By: olson Date_Written: August 22 2003 Host: zen Command: ncgen history: Wed Jul 30 08:35:58 2008: ncrename -v OZ... DODS_EXTRA.Unlimited_Dimension: time
- lat: 64
- lev: 59
- lon: 128
- time: 12
- lev(lev)float640.2842 0.3253 ... 959.0 1.004e+03
- long_name :
- hybrid level at layer midpoints (1000*(A+B))
- units :
- hybrid_sigma_pressure
- positive :
- down
array([2.84191e-01, 3.25350e-01, 3.71936e-01, 4.24615e-01, 4.84153e-01, 5.51432e-01, 6.27490e-01, 7.13542e-01, 8.11039e-01, 9.21699e-01, 1.04757e+00, 1.19108e+00, 1.35511e+00, 1.54305e+00, 1.75891e+00, 2.00739e+00, 2.29403e+00, 2.62529e+00, 3.00884e+00, 3.45369e+00, 3.97050e+00, 4.57190e+00, 5.27278e+00, 6.09073e+00, 7.04636e+00, 8.16371e+00, 9.47075e+00, 1.10000e+01, 1.27894e+01, 1.48832e+01, 1.73342e+01, 2.02039e+01, 2.35659e+01, 2.75066e+01, 3.21283e+01, 3.75509e+01, 4.39150e+01, 5.13840e+01, 6.01483e+01, 7.04289e+01, 8.24830e+01, 9.66096e+01, 1.13155e+02, 1.32514e+02, 1.55122e+02, 1.81445e+02, 2.11947e+02, 2.47079e+02, 2.87273e+02, 3.32956e+02, 3.84573e+02, 4.42610e+02, 5.07601e+02, 5.80132e+02, 6.60837e+02, 7.50393e+02, 8.49521e+02, 9.58981e+02, 1.00369e+03])
- lon(lon)float640.0 2.812 5.625 ... 354.4 357.2
- long_name :
- longitude
- units :
- degrees_east
array([ 0. , 2.8125, 5.625 , 8.4375, 11.25 , 14.0625, 16.875 , 19.6875, 22.5 , 25.3125, 28.125 , 30.9375, 33.75 , 36.5625, 39.375 , 42.1875, 45. , 47.8125, 50.625 , 53.4375, 56.25 , 59.0625, 61.875 , 64.6875, 67.5 , 70.3125, 73.125 , 75.9375, 78.75 , 81.5625, 84.375 , 87.1875, 90. , 92.8125, 95.625 , 98.4375, 101.25 , 104.0625, 106.875 , 109.6875, 112.5 , 115.3125, 118.125 , 120.9375, 123.75 , 126.5625, 129.375 , 132.1875, 135. , 137.8125, 140.625 , 143.4375, 146.25 , 149.0625, 151.875 , 154.6875, 157.5 , 160.3125, 163.125 , 165.9375, 168.75 , 171.5625, 174.375 , 177.1875, 180. , 182.8125, 185.625 , 188.4375, 191.25 , 194.0625, 196.875 , 199.6875, 202.5 , 205.3125, 208.125 , 210.9375, 213.75 , 216.5625, 219.375 , 222.1875, 225. , 227.8125, 230.625 , 233.4375, 236.25 , 239.0625, 241.875 , 244.6875, 247.5 , 250.3125, 253.125 , 255.9375, 258.75 , 261.5625, 264.375 , 267.1875, 270. , 272.8125, 275.625 , 278.4375, 281.25 , 284.0625, 286.875 , 289.6875, 292.5 , 295.3125, 298.125 , 300.9375, 303.75 , 306.5625, 309.375 , 312.1875, 315. , 317.8125, 320.625 , 323.4375, 326.25 , 329.0625, 331.875 , 334.6875, 337.5 , 340.3125, 343.125 , 345.9375, 348.75 , 351.5625, 354.375 , 357.1875])
- lat(lat)float64-87.86 -85.1 -82.31 ... 85.1 87.86
- long_name :
- latitude
- units :
- degrees_north
array([-87.8638, -85.0965, -82.3129, -79.5256, -76.7369, -73.9475, -71.1577, -68.3678, -65.5776, -62.7873, -59.997 , -57.2066, -54.4162, -51.6257, -48.8352, -46.0447, -43.2542, -40.4636, -37.6731, -34.8825, -32.0919, -29.3014, -26.5108, -23.7202, -20.9296, -18.139 , -15.3484, -12.5578, -9.7671, -6.9765, -4.1859, -1.3953, 1.3953, 4.1859, 6.9765, 9.7671, 12.5578, 15.3484, 18.139 , 20.9296, 23.7202, 26.5108, 29.3014, 32.0919, 34.8825, 37.6731, 40.4636, 43.2542, 46.0447, 48.8352, 51.6257, 54.4162, 57.2066, 59.997 , 62.7873, 65.5776, 68.3678, 71.1577, 73.9475, 76.7369, 79.5256, 82.3129, 85.0965, 87.8638])
- time(time)float644.382e+04 4.384e+04 ... 4.415e+04
- long_name :
- time
- units :
- day number (Jan 1 = 1)
- calendar :
- 365_days
array([43816., 43844., 43875., 43905., 43936., 43966., 43997., 44028., 44058., 44089., 44119., 44150.])
- P0()float64...
- long_name :
- reference pressure
- units :
- Pa
array(100369.)
- date(time)int32...
- long_name :
- current date as 8 digit integer (YYYYMMDD)
array([19900116, 19900214, 19900316, 19900415, 19900516, 19900615, 19900716, 19900816, 19900915, 19901016, 19901115, 19901216], dtype=int32)
- datesec(time)int32...
- long_name :
- seconds of current date
- units :
- s
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], dtype=int32)
- OZONE_old(time, lat, lev, lon)float64...
- long_name :
- OZONE
- units :
- FRACTION
[5799936 values with dtype=float64]
- OZONE(time, lev, lat, lon)float64...
- long_name :
- OZONE
- units :
- FRACTION
[5799936 values with dtype=float64]
- Conventions :
- NCAR-CSM
- Source :
- AMIP II (symmetric for APE project)
- Written_By :
- olson
- Date_Written :
- August 22 2003
- Host :
- zen
- Command :
- ncgen
- history :
- Wed Jul 30 08:35:58 2008: ncrename -v OZONE,OZONE_old apeozone_cam3_5_54.nc
- DODS_EXTRA.Unlimited_Dimension :
- time
Take the global average of the ozone climatology, and plot it as a function of pressure (or height)
[18]:
# Taking annual, zonal, and global averages of the ozone data
O3_zon = ozone.OZONE.mean(dim=("time","lon"))
weight_ozone = np.cos(np.deg2rad(ozone.lat)) / np.cos(np.deg2rad(ozone.lat)).mean(dim='lat')
O3_global = (O3_zon * weight_ozone).mean(dim='lat')
[19]:
O3_global.shape
[19]:
(59,)
[20]:
ax = plt.figure(figsize=(10,8)).add_subplot(111)
ax.plot( O3_global * 1.E6, np.log(O3_global.lev/const.ps) )
ax.invert_yaxis()
ax.set_xlabel('Ozone (ppm)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
yticks = np.array([1000., 500., 250., 100., 50., 20., 10., 5.])
ax.set_yticks( np.log(yticks/1000.) )
ax.set_yticklabels( yticks )
ax.set_title('Global, annual mean ozone concentration', fontsize = 24);
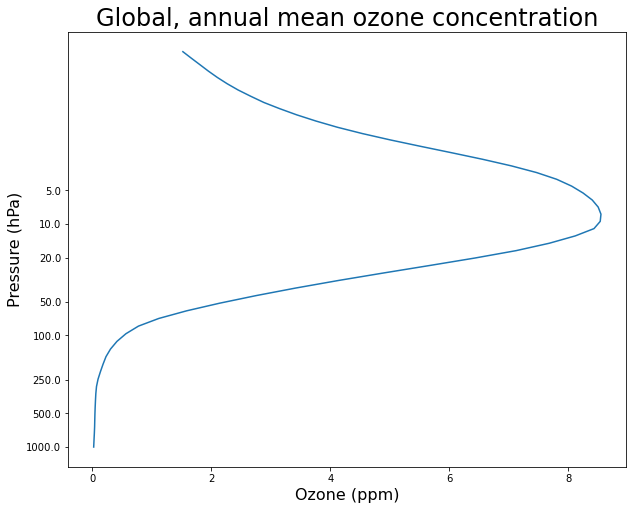
This shows that most of the ozone is indeed in the stratosphere, and peaks near the top of the stratosphere.
Now create a new column model object on the same pressure levels as the ozone data. We are also going set an adjusted lapse rate of 6 K / km, and tune the longwave absorption
[21]:
oz_col = climlab.RadiativeConvectiveModel(lev = ozone.lev,
abs_coeff=1.82E-4,
adj_lapse_rate=6,
albedo=0.315)
Now we will do something new: let the column absorb some shortwave radiation. We will assume that the shortwave absorptivity is proportional to the ozone concentration we plotted above. We need to weight the absorptivity by the pressure (mass) of each layer.
[22]:
ozonefactor = 75
dp = oz_col.Tatm.domain.axes['lev'].delta
sw_abs = O3_global * dp * ozonefactor
oz_col.subprocess.SW.absorptivity = sw_abs
oz_col.compute()
oz_col.compute()
print(oz_col.SW_absorbed_atm)
[0.01521244 0.00239547 0.00294491 0.00359022 0.00437158 0.0053308
0.006518 0.00801322 0.00983974 0.0122112 0.01517438 0.01896033
0.02378877 0.03006126 0.03813404 0.04839947 0.06121356 0.07689825
0.0956929 0.11774895 0.14311224 0.17200325 0.20535155 0.24420577
0.28873387 0.33942617 0.39635885 0.45785715 0.51545681 0.57046196
0.61908838 0.65388737 0.67529707 0.68302918 0.67627911 0.65546409
0.61861086 0.56348381 0.48940972 0.40411693 0.32861214 0.27967132
0.23974031 0.20685342 0.18078828 0.16426141 0.14274006 0.1154594
0.09590159 0.09087993 0.09138546 0.09250529 0.09439986 0.09848981
0.10256593 0.10092918 0.09452428 0.05852275 0.01312565]
Now run it out to Radiative-Convective Equilibrium, and plot
[23]:
oz_col.integrate_years(2.)
print("After " + str(oz_col.time['days_elapsed']) + " days of integration:")
print("Surface temperature is " + str(oz_col.Ts) + " K.")
print("Net energy in to the column is " + str(oz_col.ASR - oz_col.OLR) + " W / m2.")
Integrating for 730 steps, 730.4844 days, or 2.0 years.
Total elapsed time is 1.9986737567564754 years.
After 730.0 days of integration:
Surface temperature is [289.52088978] K.
Net energy in to the column is [-9.48869513e-07] W / m2.
[24]:
pozcol = oz_col.lev
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + const.tempCtoK, np.log(level/1000), 'b-', col.Tatm, np.log( pcol/const.ps ), 'r-' )
ax.plot( col.Ts, 0, 'ro', markersize=16 )
ax.plot( dalr_col.Tatm, np.log( pcol / const.ps ), 'k-' )
ax.plot( dalr_col.Ts, 0, 'ko', markersize=16 )
ax.plot( rce_col.Tatm, np.log( pcol / const.ps ), 'm-' )
ax.plot( rce_col.Ts, 0, 'mo', markersize=16 )
ax.plot( oz_col.Tatm, np.log( pozcol / const.ps ), 'c-' )
ax.plot( oz_col.Ts, 0, 'co', markersize=16 )
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/1000) )
ax.set_yticklabels( level.values )
ax.set_title('Temperature profiles: observed (blue), RE (red), dry RCE (black), moist RCE (magenta), RCE with ozone (cyan)', fontsize = 18)
ax.grid()
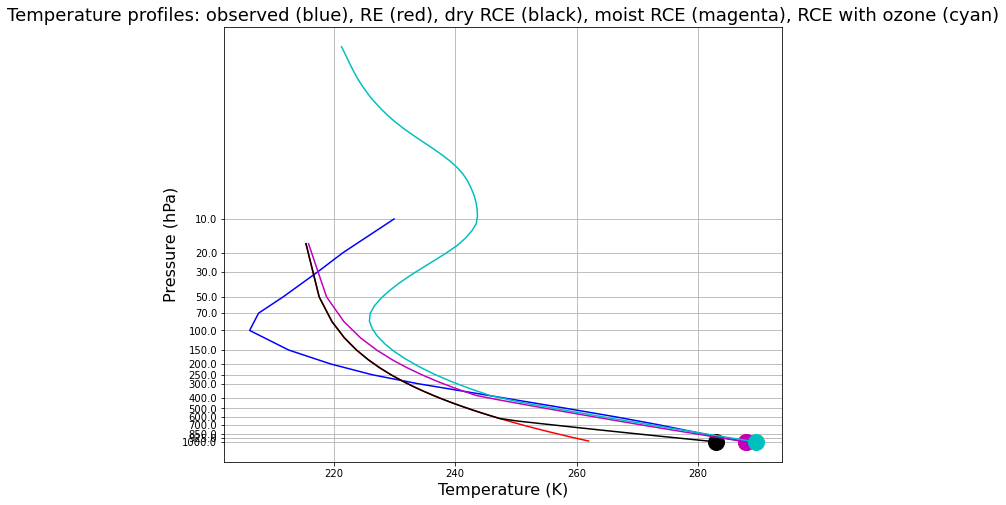
And we finally have something that looks looks like the tropopause, with temperature increasing above at about the correct rate. Though the tropopause temperature is off by 15 degrees or so.
Greenhouse warming in the RCE model with ozone¶
[25]:
oz_col2 = climlab.process_like( oz_col )
[26]:
oz_col2.subprocess['LW'].absorptivity *= 1.2
[27]:
oz_col2.integrate_years(2.)
Integrating for 730 steps, 730.4844 days, or 2.0 years.
Total elapsed time is 3.997347513512951 years.
[28]:
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + const.tempCtoK, np.log(level/const.ps), 'b-' )
ax.plot( oz_col.Tatm, np.log( pozcol / const.ps ), 'c-' )
ax.plot( oz_col.Ts, 0, 'co', markersize=16 )
ax.plot( oz_col2.Tatm, np.log( pozcol / const.ps ), 'c--' )
ax.plot( oz_col2.Ts, 0, 'co', markersize=16 )
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/const.ps) )
ax.set_yticklabels( level.values )
ax.set_title('Temperature profiles: observed (blue), RCE with ozone (cyan)', fontsize = 18)
ax.grid()
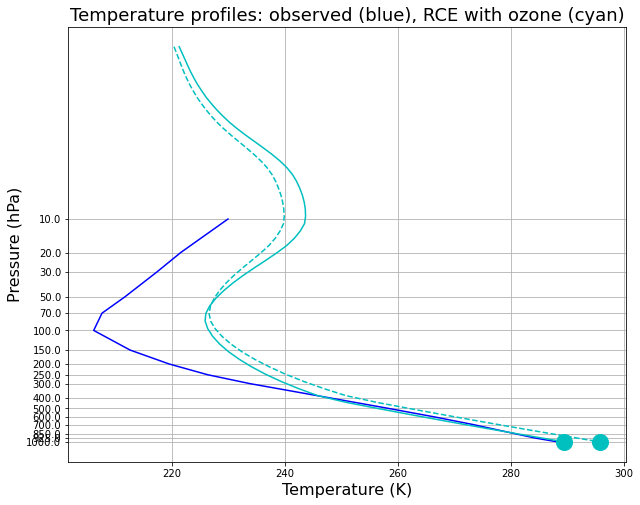
And we find that the troposphere warms, while the stratosphere cools!
Vertical structure of greenhouse warming in CESM model¶
[29]:
datapath = "http://thredds.atmos.albany.edu:8080/thredds/dodsC/CESMA/"
atmstr = ".cam.h0.clim.nc"
cesm_ctrl = xr.open_dataset(datapath + 'som_1850_f19/clim/som_1850_f19' + atmstr)
cesm_2xCO2 = xr.open_dataset(datapath + 'som_1850_2xCO2/clim/som_1850_2xCO2' + atmstr)
[30]:
cesm_ctrl.T
[30]:
<xarray.DataArray 'T' (time: 12, lev: 26, lat: 96, lon: 144)> [4313088 values with dtype=float32] Coordinates: * lev (lev) float64 3.545 7.389 13.97 23.94 ... 867.2 929.6 970.6 992.6 * time (time) object 0001-01-15 00:00:00 ... 0001-12-15 00:00:00 * lat (lat) float64 -90.0 -88.11 -86.21 -84.32 ... 84.32 86.21 88.11 90.0 * lon (lon) float64 0.0 2.5 5.0 7.5 10.0 ... 350.0 352.5 355.0 357.5 Attributes: mdims: 1 units: K long_name: Temperature cell_methods: time: mean time: mean
- time: 12
- lev: 26
- lat: 96
- lon: 144
- ...
[4313088 values with dtype=float32]
- lev(lev)float643.545 7.389 13.97 ... 970.6 992.6
- long_name :
- hybrid level at midpoints (1000*(A+B))
- units :
- level
- positive :
- down
- standard_name :
- atmosphere_hybrid_sigma_pressure_coordinate
- formula_terms :
- a: hyam b: hybm p0: P0 ps: PS
array([ 3.544638, 7.388814, 13.967214, 23.944625, 37.23029 , 53.114605, 70.05915 , 85.439115, 100.514695, 118.250335, 139.115395, 163.66207 , 192.539935, 226.513265, 266.481155, 313.501265, 368.81798 , 433.895225, 510.455255, 600.5242 , 696.79629 , 787.70206 , 867.16076 , 929.648875, 970.55483 , 992.5561 ])
- time(time)object0001-01-15 00:00:00 ... 0001-12-...
- long_name :
- time
- bounds :
- time_bnds
- cell_methods :
- time: mean
array([cftime.DatetimeNoLeap(1, 1, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 2, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 3, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 4, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 5, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 6, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 7, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 8, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 9, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 10, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 11, 15, 0, 0, 0, 0), cftime.DatetimeNoLeap(1, 12, 15, 0, 0, 0, 0)], dtype=object)
- lat(lat)float64-90.0 -88.11 -86.21 ... 88.11 90.0
- long_name :
- latitude
- units :
- degrees_north
array([-90. , -88.105263, -86.210526, -84.315789, -82.421053, -80.526316, -78.631579, -76.736842, -74.842105, -72.947368, -71.052632, -69.157895, -67.263158, -65.368421, -63.473684, -61.578947, -59.684211, -57.789474, -55.894737, -54. , -52.105263, -50.210526, -48.315789, -46.421053, -44.526316, -42.631579, -40.736842, -38.842105, -36.947368, -35.052632, -33.157895, -31.263158, -29.368421, -27.473684, -25.578947, -23.684211, -21.789474, -19.894737, -18. , -16.105263, -14.210526, -12.315789, -10.421053, -8.526316, -6.631579, -4.736842, -2.842105, -0.947368, 0.947368, 2.842105, 4.736842, 6.631579, 8.526316, 10.421053, 12.315789, 14.210526, 16.105263, 18. , 19.894737, 21.789474, 23.684211, 25.578947, 27.473684, 29.368421, 31.263158, 33.157895, 35.052632, 36.947368, 38.842105, 40.736842, 42.631579, 44.526316, 46.421053, 48.315789, 50.210526, 52.105263, 54. , 55.894737, 57.789474, 59.684211, 61.578947, 63.473684, 65.368421, 67.263158, 69.157895, 71.052632, 72.947368, 74.842105, 76.736842, 78.631579, 80.526316, 82.421053, 84.315789, 86.210526, 88.105263, 90. ])
- lon(lon)float640.0 2.5 5.0 ... 352.5 355.0 357.5
- long_name :
- longitude
- units :
- degrees_east
array([ 0. , 2.5, 5. , 7.5, 10. , 12.5, 15. , 17.5, 20. , 22.5, 25. , 27.5, 30. , 32.5, 35. , 37.5, 40. , 42.5, 45. , 47.5, 50. , 52.5, 55. , 57.5, 60. , 62.5, 65. , 67.5, 70. , 72.5, 75. , 77.5, 80. , 82.5, 85. , 87.5, 90. , 92.5, 95. , 97.5, 100. , 102.5, 105. , 107.5, 110. , 112.5, 115. , 117.5, 120. , 122.5, 125. , 127.5, 130. , 132.5, 135. , 137.5, 140. , 142.5, 145. , 147.5, 150. , 152.5, 155. , 157.5, 160. , 162.5, 165. , 167.5, 170. , 172.5, 175. , 177.5, 180. , 182.5, 185. , 187.5, 190. , 192.5, 195. , 197.5, 200. , 202.5, 205. , 207.5, 210. , 212.5, 215. , 217.5, 220. , 222.5, 225. , 227.5, 230. , 232.5, 235. , 237.5, 240. , 242.5, 245. , 247.5, 250. , 252.5, 255. , 257.5, 260. , 262.5, 265. , 267.5, 270. , 272.5, 275. , 277.5, 280. , 282.5, 285. , 287.5, 290. , 292.5, 295. , 297.5, 300. , 302.5, 305. , 307.5, 310. , 312.5, 315. , 317.5, 320. , 322.5, 325. , 327.5, 330. , 332.5, 335. , 337.5, 340. , 342.5, 345. , 347.5, 350. , 352.5, 355. , 357.5])
- mdims :
- 1
- units :
- K
- long_name :
- Temperature
- cell_methods :
- time: mean time: mean
[31]:
T_cesm_ctrl_zon = cesm_ctrl.T.mean(dim=('time', 'lon'))
T_cesm_2xCO2_zon = cesm_2xCO2.T.mean(dim=('time', 'lon'))
[32]:
weight = np.cos(np.deg2rad(cesm_ctrl.lat)) / np.cos(np.deg2rad(cesm_ctrl.lat)).mean(dim='lat')
T_cesm_ctrl_glob = (T_cesm_ctrl_zon*weight).mean(dim='lat')
T_cesm_2xCO2_glob = (T_cesm_2xCO2_zon*weight).mean(dim='lat')
[33]:
fig = plt.figure( figsize=(10,8) )
ax = fig.add_subplot(111)
ax.plot( Tglobal + const.tempCtoK, np.log(level/const.ps), 'b-' )
ax.plot( oz_col.Tatm, np.log( pozcol / const.ps ), 'c-' )
ax.plot( oz_col.Ts, 0, 'co', markersize=16 )
ax.plot( oz_col2.Tatm, np.log( pozcol / const.ps ), 'c--' )
ax.plot( oz_col2.Ts, 0, 'co', markersize=16 )
ax.plot( T_cesm_ctrl_glob, np.log( cesm_ctrl.lev/const.ps ), 'r-' )
ax.plot( T_cesm_2xCO2_glob, np.log( cesm_ctrl.lev/const.ps ), 'r--' )
ax.invert_yaxis()
ax.set_xlabel('Temperature (K)', fontsize=16)
ax.set_ylabel('Pressure (hPa)', fontsize=16 )
ax.set_yticks( np.log(level/const.ps) )
ax.set_yticklabels( level.values )
ax.set_title('Temperature profiles: observed (blue), RCE with ozone (cyan), CESM (red)', fontsize = 18)
ax.grid()
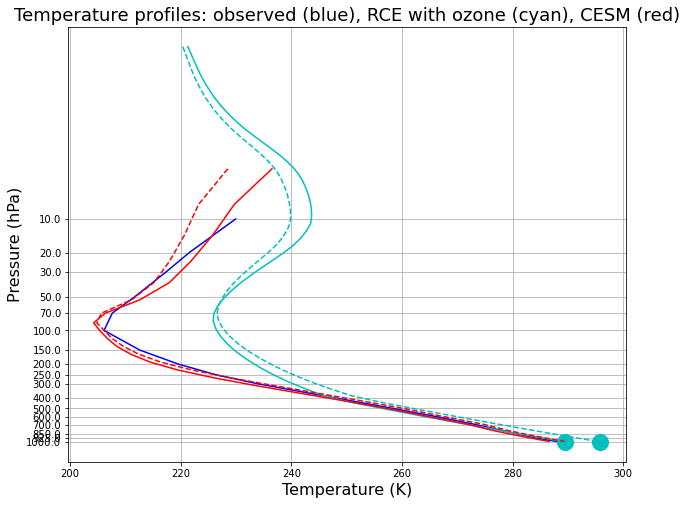
And we find that CESM has the same tendency for increased CO2: warmer troposphere, colder stratosphere.
[ ]: